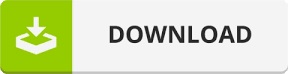
To permute both rows and columns I think you either have to run it twice, or pull some ugly shenanigans with numpy.unravel_index that hurts my head to think about. PVec0, pVec1 = calcMyPermutationVectors()Īrr.take(pVec0, axis=0, out=arr, mode="clip")Īrr.take(pVec1, axis=1, out=arr, mode="clip") So in total you call should look something like the following: #Inplace Rearrange On a final note, take seems to be a array method, so instead of np.take(i, rr, axis=0) If you don't set the mode it will make a copy to restore the array state on a Python exception (see here). To do it in-place, all you need to do is specify the "out" parameter to be the same as the input array AND you have to set the mode="clip" or mode="wrap". If you are looking for a method that accepts multiple arrays together and shuffles them, then there exists one in the scikit-learn package. Here is an example of doing a random permutation of an identity matrix's rows: import numpy as npĪrray(, We are first generating a random permutation of the integer values in the range 0, len(x)), and then using the same to index the two arrays. You can do this in-place with numpy's take() function, but it requires a bit of hoop jumping.
NUMPY PERMUTE FULL
Warning: The below example works properly, but using the full set of parameters suggested at the post end exposes a bug, or at least an "undocumented feature" in the numpy.take() function. Mathematically this corresponds to pre-multiplying the matrix by the permutation matrix P and post-multiplying it by P-1 PT, but this is not a computationally reasonable solution. I currently copy matrices this large unnecessarily, but I would hope this could be easily avoided for permutation. 31 I want to modify a dense square transition matrix in-place by changing the order of several of its rows and columns, using python's numpy library. Actually I would like to use matrices as large as possible, but I don't want to deal with the inconvenience of not being able to hold them in RAM, and I do O(N^3) LAPACK operations on the matrix which would also limit the practical matrix size. The matrix is small enough to fit into desktop RAM but big enough that I do not want to copy it thoughtlessly. Or they mean counting or enumerating all possible permutations. Sometimes when people talk about permutations, they only mean the sampling of random permutations, for example as part of a procedure to obtain p-values in statistics.
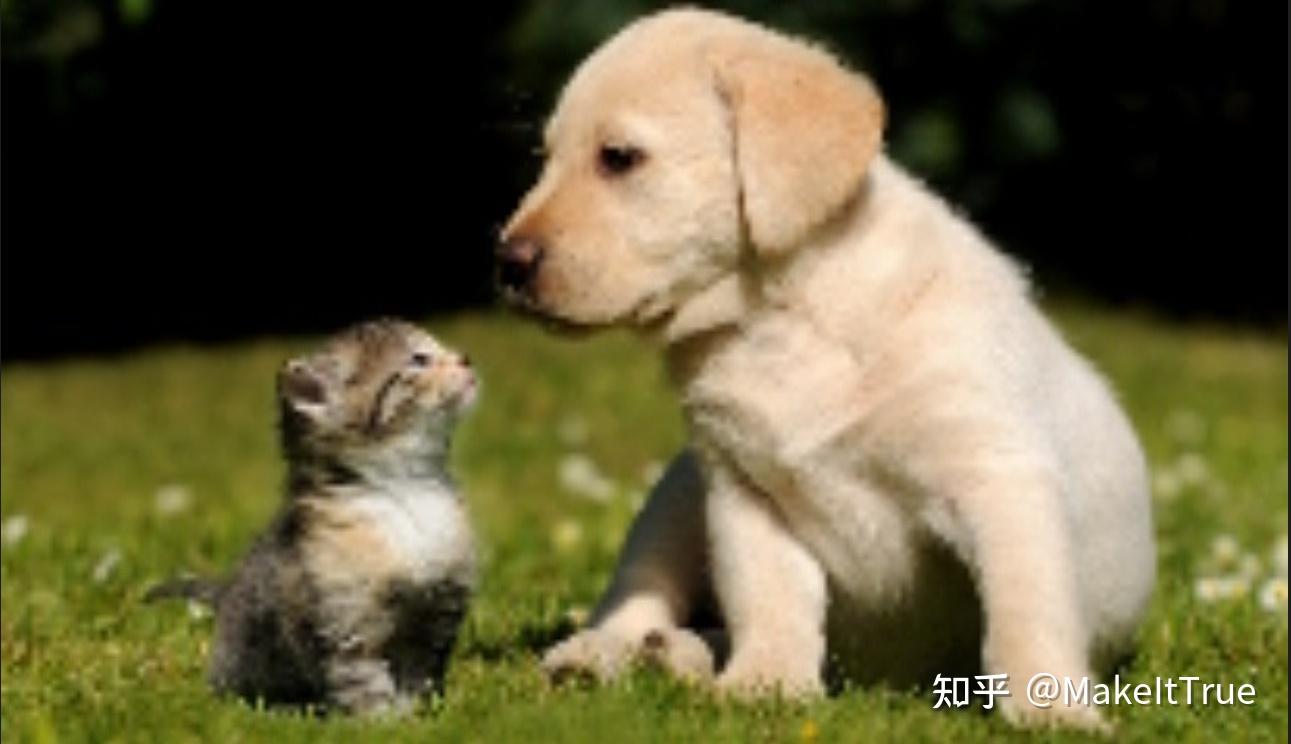
Also for some simple situations it may be sufficient to just separately track an index permutation, but this is not convenient in my case. Something like this might be possible with numpy's "advanced indexing" but my understanding is that such a solution would not be in-place. Maybe I am just failing at searching the internet.
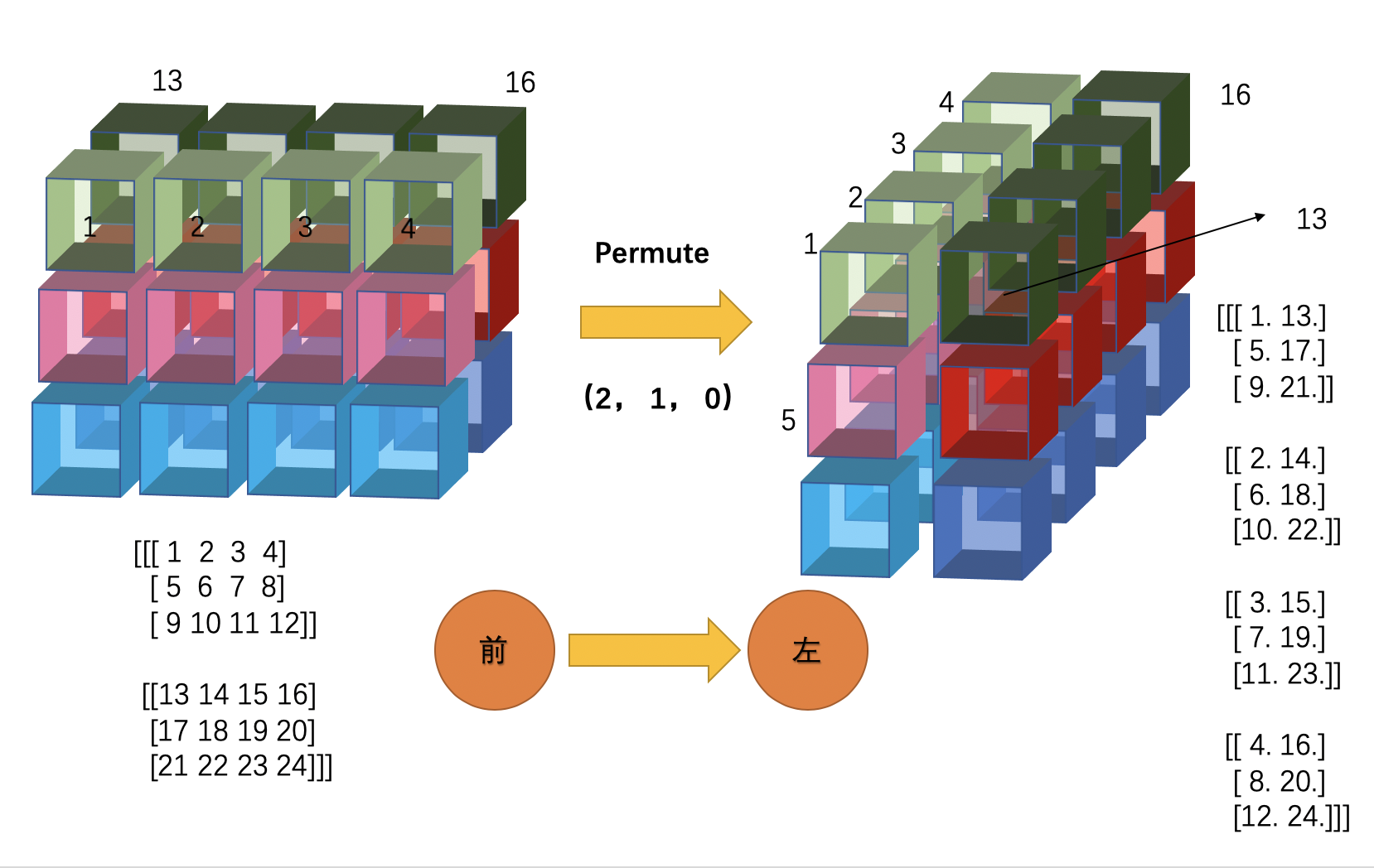
Right now I am manually swapping rows and columns, but I would have expected numpy to have a nice function f(M, v) where M has n rows and columns, and v has n entries, so that f(M, v) updates M according to the index permutation v. Mathematically this corresponds to pre-multiplying the matrix by the permutation matrix P and post-multiplying it by P^-1 = P^T, but this is not a computationally reasonable solution. I want to modify a dense square transition matrix in-place by changing the order of several of its rows and columns, using python's numpy library.
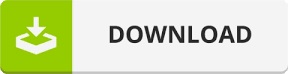